iOS
This page explains how to configure push notifications for iOS devices. Push notifications are a simple and effective way to increase engagement and communicate with your customers.
1. Enable Push Notifications on Apple Developer Account
Follow these steps to create a development and production push notification SSL certificates.
- Log into the Apple Developer Member Center.
- Click on Certificates, Identifiers & Profiles.
- Next click the Identifiers folder under iOS Apps.
- Then select the app that will be receiving the notifications.
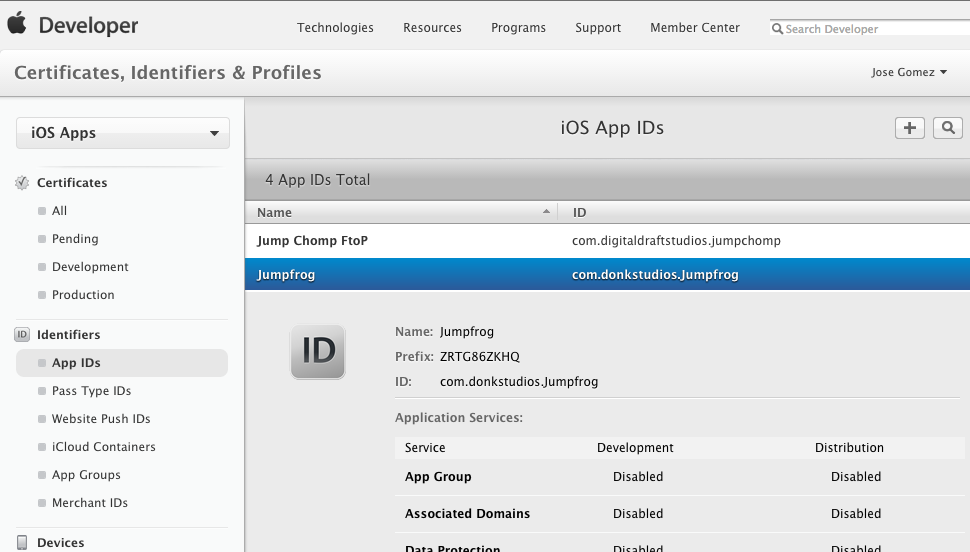
app identifiers
If push notifications is not enabled click edit, check the push notification box and create the certificates. Once the certificates are created make sure they are installed on the mac being used to develop by downloading them and double-clicking.
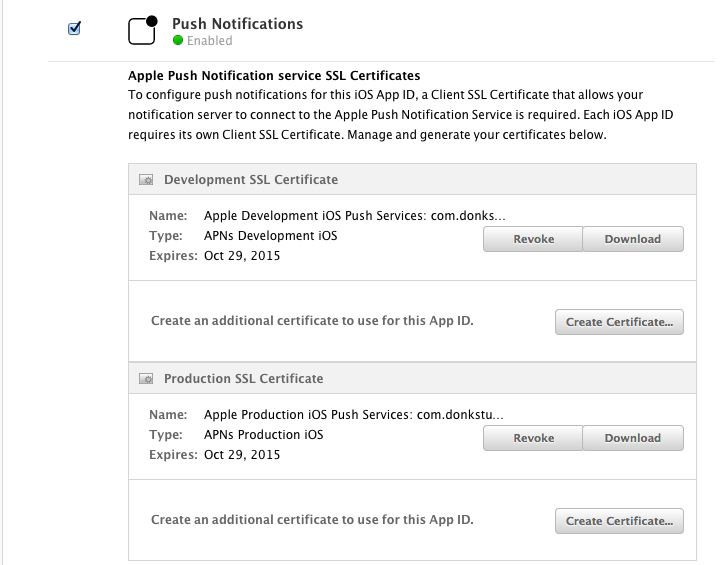
push notification settings
Once the certificates have been installed open Keychain Access and right-click on the certificate to export to a .p12 file. The password can be left empty. This is the file that should be uploaded into the Vessel.io campaign certificate form.
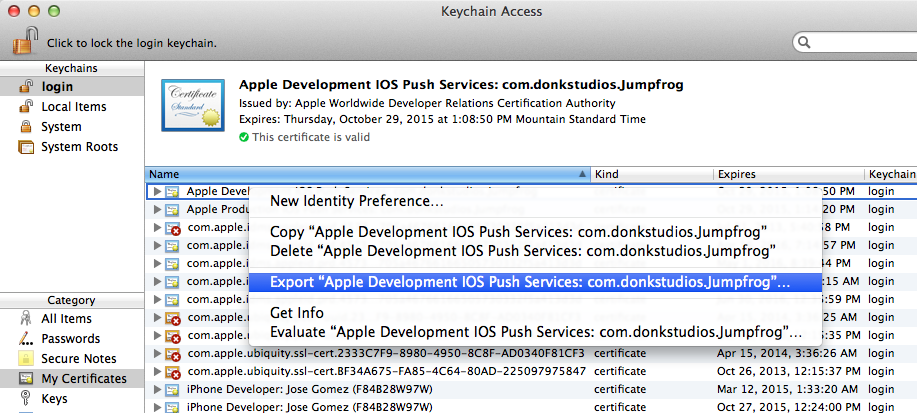
keychain access
The last step is to recreate all provisioning files.
2. Enable Push Notification
Add the following code to the App delegate in order to deliver push notifications on your customer's devices.
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
Vessel *sharedInstance = [Vessel sharedInstance];
[sharedInstance initializeWithAppSecret:@"AppSecret" launchOptions:launchOptions]
...
// Register for Push Notitications, if running iOS 8
if ([application respondsToSelector:@selector(registerUserNotificationSettings:)]) {
UIUserNotificationType userNotificationTypes = (UIUserNotificationTypeAlert |
UIUserNotificationTypeBadge |
UIUserNotificationTypeSound);
UIUserNotificationSettings *settings = [UIUserNotificationSettings settingsForTypes:userNotificationTypes
categories:nil];
[application registerUserNotificationSettings:settings];
[application registerForRemoteNotifications];
} else {
// Register for Push Notifications before iOS 8
[application registerForRemoteNotificationTypes:(UIRemoteNotificationTypeBadge |
UIRemoteNotificationTypeAlert |
UIRemoteNotificationTypeSound)];
}
...
}
"YOUR_APP_NAME" Would Like to Send You Push Notifications
Call this method to initiate the registration process with Apple Push Service. If registration succeeds, the app calls your App delegate object’s application:didRegisterForRemoteNotificationsWithDeviceToken: method and passes it a device token.
Failed to Register Push Notification
If registration fails, the app calls its App delegate’s application:didFailToRegisterForRemoteNotificationsWithError: method instead.
3. Register Push Token with Vessel
In order to receive push notifications from Vessel. You need to register the device token with Vessel.
-(void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken{
// Register Push Notification
[[Vessel sharedInstance] registerPushDeviceToken:deviceToken];
}
4. Track Push Notifications
App Running in Background.
If the App is running in the background or not active then the device will receive a push notification as shown bellow. Vessel will track the open in this case.
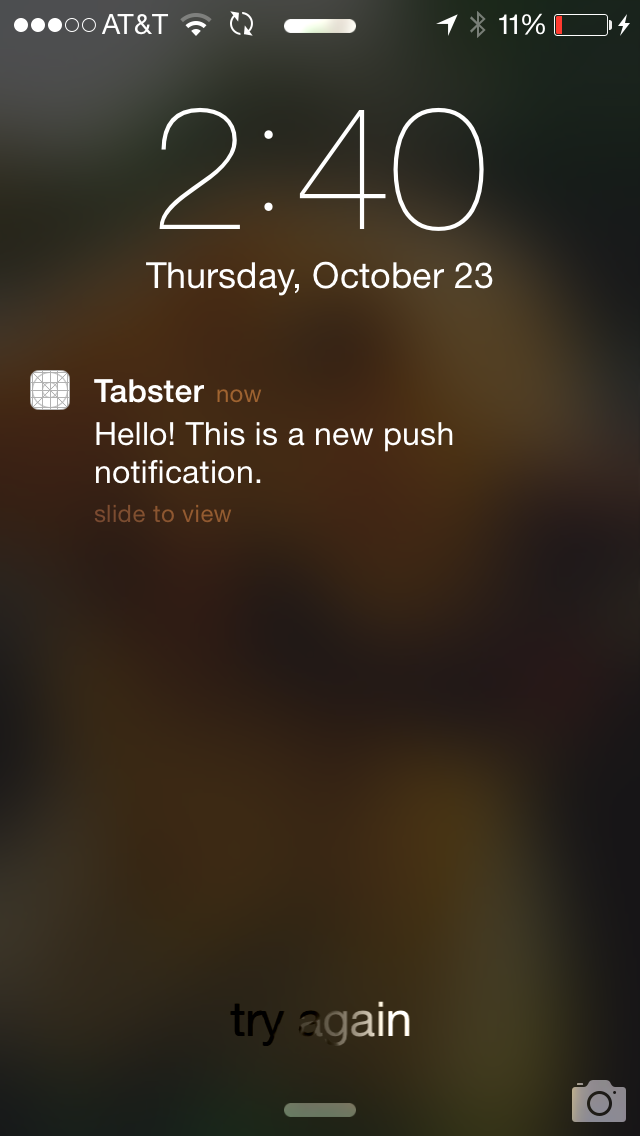
App Running in Foreground
If device receives a push notification while the App is running in foreground, it will not show standard push notification. Instead it will be passed to application:didReceiveRemoteNotification: callback on your App delegate, You can pass this callback to Vessel to handle as shown bellow.
- (void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo{
// Depending upon configuration you have selected while composing a push notification, Vessel will show standard alert message as shown bellow
[[Vessel sharedInstance] handlePushNotification:userInfo];
// or if you want to manually track open event then you can call
// [[Vessel sharedInstance] trackPushNotification:userInfo]
}
Interrupt when user active
While composing a push notification if you enabled this flag and if user is active when the push notification is delivered, Vessel will provide an alert message as show below.
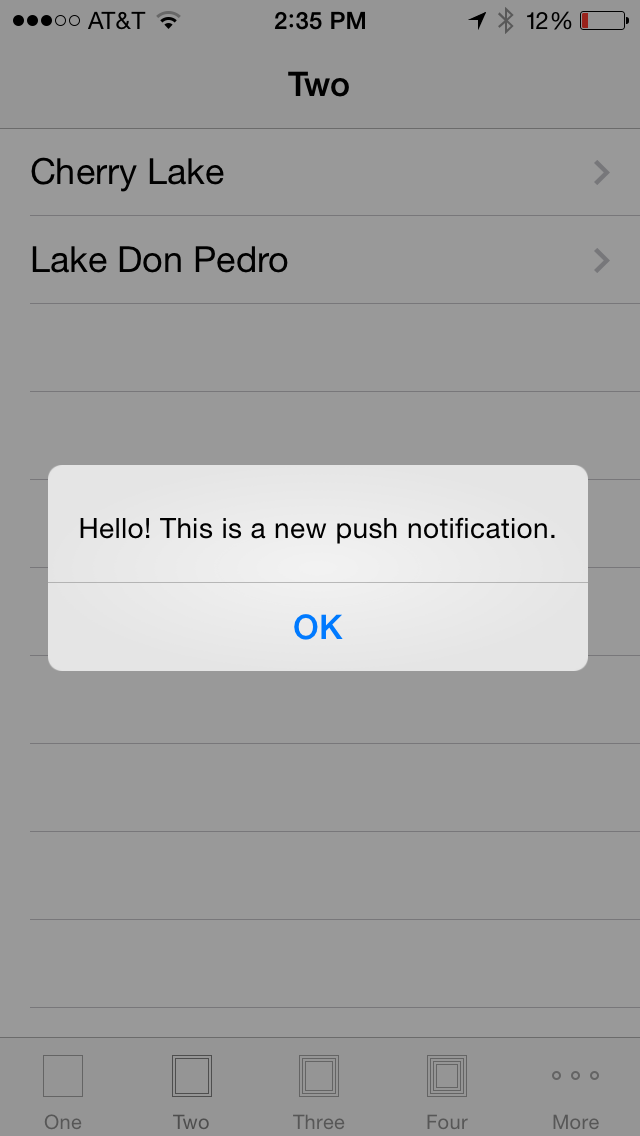
Updated less than a minute ago